My Kratky tomato project, tracking a Kratky setup from start to finish
Fully passive, hydroponic setups are now everywhere. However, it seems no one has taken the time to diligently record how the nutrient solution changes through time in these setups and what problems these changes can generate for plant growth. In my Kratky tomato project, I will be closely monitoring a completely passive Kratky setup from start to finish. In this post, I will describe how this project will work, what I will be recording, and what I’m hoping to achieve. Check out the youtube video below for an initial intro to this project.
The goals
It is tough to grow large flowering plants using truly passive Kratky setups (read my blog post on the matter). We know this is because of issues related to their increased water uptake and the large nutrient and pH imbalances these plants create in nutrient solutions. However, I haven’t found any data set that shows how these problems develop as a function of time. By measuring different variables in a Kratky setup through an entire crop cycle, I hope to gather data to help us understand what goes wrong, why it goes wrong and when it goes wrong. With this information, we should be able to develop better nutrient solutions and management techniques, for more successful Kratky hydroponic setups for large flowering plants.
The setup
The setup is a 13L bucket wrapped in duct tape – to prevent light from entering the system – with a hole at the top and a net pot containing a tomato plant. The tomato – which I have named Bernard – is an indeterminate cherry tomato that was germinated in the net pot. The net pot contains a medium consisting of 50% rice hulls and 50% river sand. The bucket has been filled with a store-bought generic hydroponic nutrient solution up to the point where it touches the bottom of the net pot. Furthermore, the bucket is placed inside a grow tent and receives 12 hours of light from a Mars Hydro TS 600 Full Spectrum lamp. The light has been initially placed around 10 inches above the plant and will be moved as needed to maintain proper leaf temperature and light coverage of the plant.
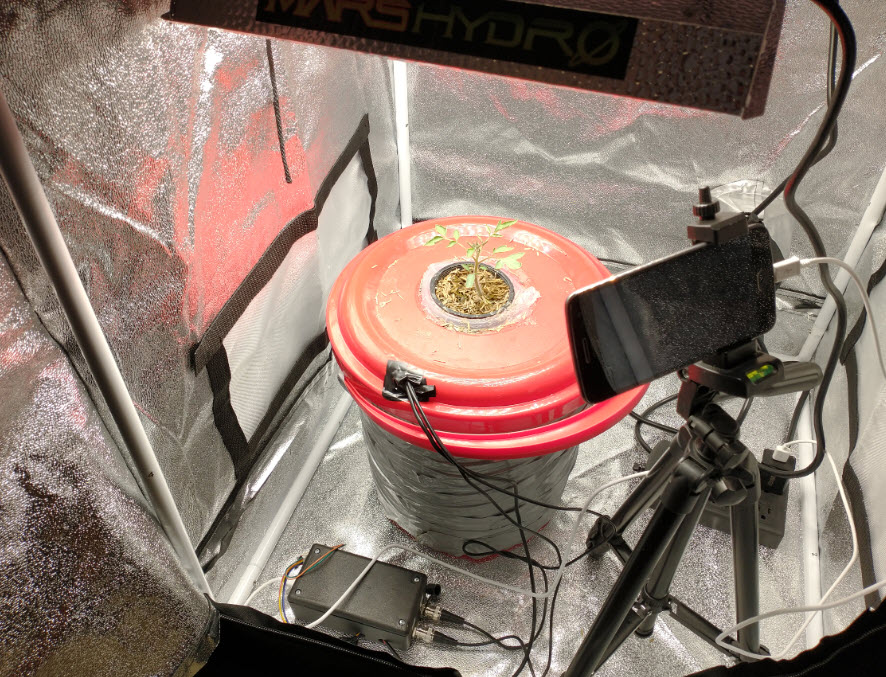
The measurements
I will be monitoring as many variables as I can within this experiment. To do this I have set up an Arduino MKR Wifi 1010 that uses self-isolated uFire pH and EC probes, a BME280 sensor to monitor air temperature and humidity, and a DS18B20 sensor to monitor the temperature of the solution. I will also be using Horiba probes to track the Nitrate, Potassium, and Calcium concentrations once per day. All the Arduino’s readings are being sent via Wifi to a MyCodo server in a Raspberry Pi, using the MQTT messaging protocol. The data is then recorded into the MyCodo’s database and also displayed in a custom dashboard. The ISE measurements are manually recorded on a spreadsheet.
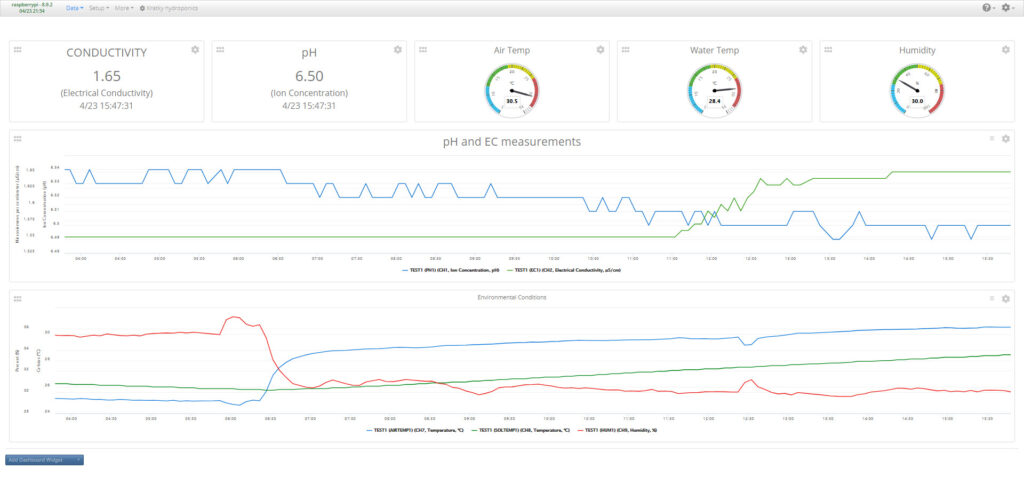
Furthermore, I am also taking photographs every 15 minutes – when the lights are on – using a smartphone. This will allow me to create a time-lapse showing the growth of the plant from the very early seedling to late fruiting stages.
Conclusion
I have started a new project where I will fully record the complete development process of a large flowering plant in a Kratky setup. We will have information about the EC and pH changes of the solution, as well as information about how different nutrient concentrations (N, K and Ca) change through the life of the plant. With this information, we should be able to figure out how to modify the nutrient solution to grow large flowering plants more successfully, and what interventions might be critical in case fully passive growth is not possible.
I will continue to share updates of this project in both my blog and YouTube channel.
What do you think about this project? Do you think Bernard will make it? Let us know in the comments below!